2 min to read
Java Top-level Access Control
A concise guide to mastering Java top-level access control
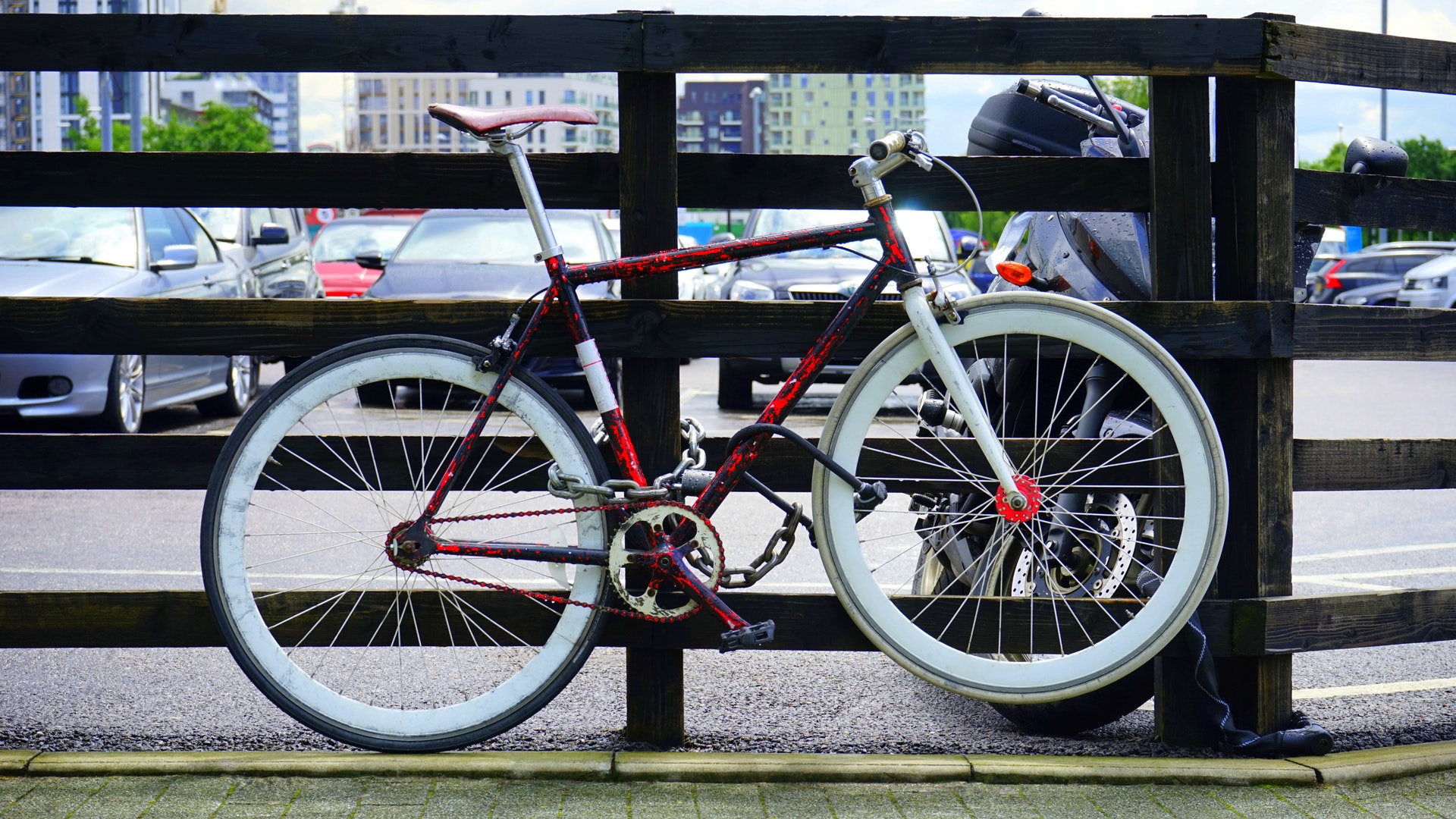
Welcome.
Today’s post is about Java access control, an important aspect of Object Oriented Design. If you want to go in-depth into class design and best practices, encapsulation is the building block. So let’s get started.
Top-level
When we speak of access control in Java all you have to understand is that it all depends on:
- Where the entity’s definition is and
- Which access modifier it was used.
Definition
Top-level: Class or interface that is not nested in other class or interface.
Follow below a table with the rules before I explain.
Access Control | Modifier | Accessible |
---|---|---|
Public | public | Everywhere |
Package-private | Within the same package |
If you want to access a class/interface, you can do it:
- With public modifier: From any package.
- Without modifier (Package-private): Just from within the same package.
Easy, isn’t it? I’m going to show you an example.
package client;
import server.Server;
class Client {
private Server server;
public Client(Server server) {
this.server = server;
}
public boolean checkServerUp() {
return server.isUp();
}
}
package server;
public class Server {
private Connection conn;
public Server(Connection conn) {
this.conn = conn;
}
public boolean isUp() {
return conn.connect();
}
}
Did you see that? The Client can import and use the Server even though they’re in different packages because the Server is defined as public. The opposite is not true. The Server couldn’t reach the Client because the Client has no modifier (implies Private-package modifier) and they’re in different packages. If the Client would be in the same package as the Server is could be possible.
Thanks,
Eduardo Augusto Benigno da Silva
Comments